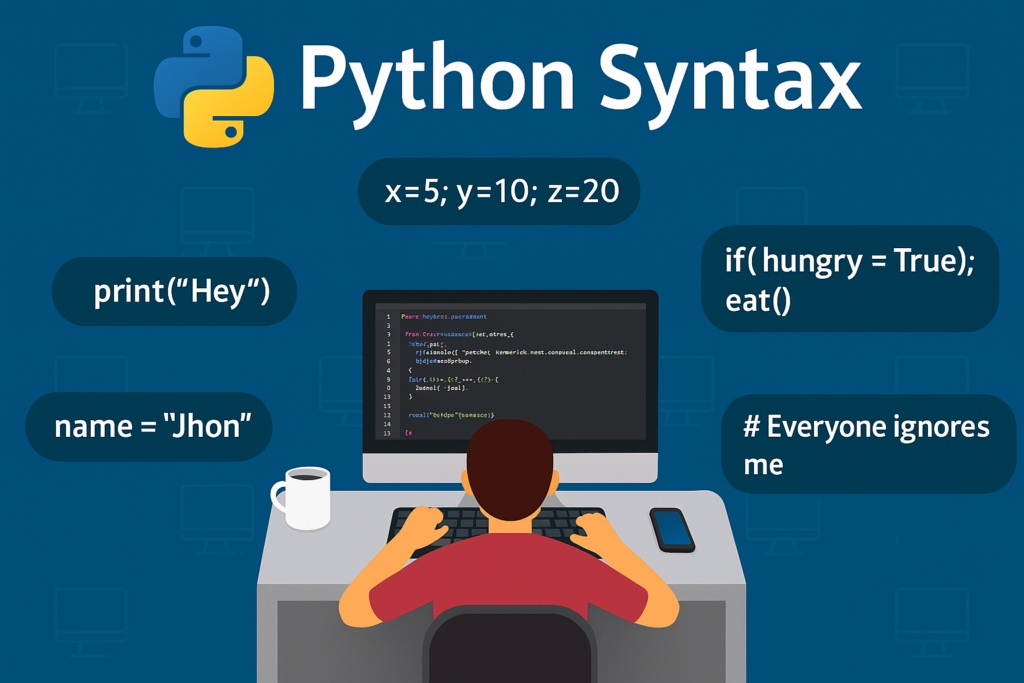
Contents
Python Syntax
If you're just starting your Python programming learning journey, you've come to the right place. Python is one of the simplest and most popular programming languages currently — and what makes it so popular is its clean and readable syntax.
In this blog, we’ll take you step-by-step through the basics of Python syntax in a way that's super easy to understand. Whether you’re a school student, a college beginner, or someone completely new to coding — this guide is perfect for you.
📌 What Is Python Syntax?
Python syntax refers to the set of rules that define how a Python program is written and interpreted. It’s like grammar in a language — if you follow the rules, your code works. If not, Python will throw an error.
🐍 Why Python Syntax Is So Easy?
- No need to add semicolons (
;
) at the end of each line. - No curly braces
{}
like in C or Java. - Uses indentation to define code blocks.
- Syntax looks like plain English!
✅ Let's Dive into Python Syntax Basics
1️⃣ Printing Output
print("Hello, World!")
Output:
Hello, World!
2️⃣ Comments in Python
Comment can be useful in writing in-code documentation for your future refrence. There are mainly two type of comment. We will discuss comment in future Tutorial in much details.
Single-line comment:
# This is a comment
print("Python is fun!")
Multi-line comment:
'''
This is a
multi-line comment
'''
print("Learning Python!")
3️⃣ Variables and Assignment
name = "Ankit"
age = 24
height = 171.5
print(name)
print(age)
print(height)
4️⃣ Indentation in Python
if age > 18:
print("You are an adult")
❌ Wrong Indentation will give an error.
5️⃣ Data Types
Data Type | Example |
---|---|
int | 10 |
float | 10.5 |
str | "Hello" |
bool | True, False |
list | [1, 2, 3] |
tuple | (4, 5, 6) |
dict | {"a": 1} |
x = 10
print(type(x))
6️⃣ Taking User Input
name = input("Enter your name: ")
print("Hello, " + name + "!")
💡 Note: input() always returns a string
.
7️⃣ Conditional Statements (if, elif, else)
age = 20
if age < 18:
print("Minor")
elif age == 18:
print("Just 18")
else:
print("Adult")
8️⃣ Loops in Python
While Loop:
i = 1
while i <= 5:
print(i)
i += 1
For Loop:
for i in range(1, 6):
print(i)
9️⃣ Functions
Simple function:
def greet():
print("Hello from function!")
greet()
Function with parameters:
def add(a, b):
print(a + b)
add(5, 3)
🔟 Python Is Case-Sensitive
name = "Ankit"
Name = "Goswami"
print(name) # prints "Ankit"
print(Name) # prints "Goswami"
These are two different variables.
📋 Summary of Key Points
Concept | Example |
---|---|
Printing | print("Hi") |
Comment | # This is a comment |
Variable | x = 10 |
Indentation | if x > 5: |
Data Types | int, str, float, bool, list... |
User Input | input("Enter name: ") |
Condition | if, elif, else |
Loops | for, while |
Functions | def myFunction(): |
Case Sensitivity | name != Name |
🎯 Final Words
Learning Python syntax is like learning the alphabet before writing essays. Once you master the basic rules, you can move on to writing real programs, building apps, and even diving into machine learning or web development.
Don’t worry if you make mistakes — everyone does when they start out. Just keep practicing, and soon you'll be writing Python code with confidence!
🧠 Want to Practice?
Try writing a small program that:
- Asks for your name
- Greets you
- Asks your age
- Tells if you're eligible to vote (age ≥ 18)
Need help? Drop a comment below or visit us at TechieTrend!